Add multiple filters to current view
This script will add filters from Revit project to current view with visibility control option.
Script scheme is:
"View & it name" node code is:
"WindowsForm Create" node code is:
"Add flters" node code is:
"Show message" node code is:
Download script for Dynamo - Link
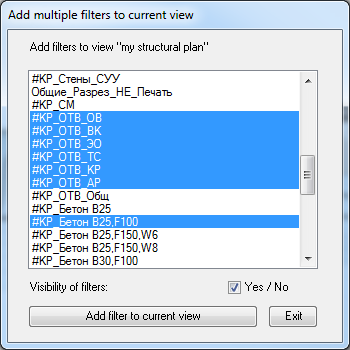
Script scheme is:
"View & it name" node code is:
import clr clr.AddReference("RevitServices") import RevitServices from RevitServices.Persistence import DocumentManager doc = DocumentManager.Instance.CurrentDBDocument myview = doc.ActiveView myviewname = myview.Name OUT = myviewname, myview
"WindowsForm Create" node code is:
import clr clr.AddReference('System.Windows.Forms') clr.AddReference('System.Drawing') from System.Drawing import * from System.Windows.Forms import * class TESForm(Form): def __init__(self, args, viewname): viewlen = len(viewname)*15 self.Text = "Add multiple filters to current view" self.Width = viewlen + 80 self.Height = 350 self.MaximizeBox = False self.MinimizeBox = False self.ControlBox = False self.FormBorderStyle = FormBorderStyle.FixedSingle self.ShowIcon = False self.StartPosition = FormStartPosition.CenterScreen self.TopMost = True self.values = [] header = 'Add filters to view "%s"' %viewname self.label = Label(Text = header) self.label.Location = Point(20, 10) self.label.Height = 20 self.label.Width = viewlen + 30 self.list = ListBox() self.list.Size = Size(viewlen + 20, 200) self.list.Location = Point(20, 40) for i in args: self.list.Items.Add(i.Name) self.list.SelectionMode = SelectionMode.MultiExtended self.list.SetSelected(0, True) self.label_n = Label(Text = "Visibility of filters:") self.label_n.Location = Point(20, 250) self.label_n.Height = 20 self.label_n.Width = 200 self.check = CheckBox() self.check.Location = Point(220, 246) self.check.Text = 'Yes / No' self.check.Checked = True self.check.Width = 80 self.button = Button() self.button.Text = "Add filter to current view" self.button.Width = viewlen + 20 - 60 self.button.Location = Point (20, 275) self.button.Click += self.setclose self.button1 = Button() self.button1.Text = "Exit" self.button1.Width = 50 self.button1.Location = Point (viewlen + 20 - 30, 275) self.button1.Click += self.setexit self.Controls.Add(self.label) self.Controls.Add(self.button) self.Controls.Add(self.button1) self.Controls.Add(self.list) self.Controls.Add(self.label_n) self.Controls.Add(self.check) def setclose(self, sender, event): sub = form.list.SelectedIndices, form.list.SelectedItems sup = form.check.Checked self.values = sub, sup, 'Ok' self.Close() def setexit(self, sender, event): sub = [], [] self.values = sub, False, 'Exit' self.Close() viewfilters = IN[0] current_view = IN[1][0] form = TESForm(viewfilters, current_view) form.ShowDialog() result = list(form.values[0]) Indices = result[0] viewfilterstoadd = [viewfilters[idx] for idx in Indices] OUT = viewfilterstoadd, form.values[1], form.values[2]
"Add flters" node code is:
import clr clr.AddReference("RevitServices") import RevitServices from RevitServices.Persistence import DocumentManager from RevitServices.Transactions import TransactionManager doc = DocumentManager.Instance.CurrentDBDocument clr.AddReference("RevitAPI") import Autodesk from Autodesk.Revit.DB import * import System from System.Collections.Generic import * dataEnteringNode = IN filters = UnwrapElement(IN[0][0]) view = UnwrapElement(IN[1][1]) visOrNot = IN[0][1] output = [] TransactionManager.Instance.EnsureInTransaction(doc) for fil in filters: try: view.AddFilter(fil.Id) view.SetFilterVisibility(fil.Id, visOrNot) output.append(fil.Name) except: pass TransactionManager.Instance.TransactionTaskDone() OUT = output
"Show message" node code is:
import clr clr.AddReference('RevitAPIUI') from Autodesk.Revit.UI import * dataEnteringNode = IN name = IN[2][0] filtersnames = IN[0] if len(filtersnames) != 0: maincontent = u'\n'.join(filtersnames) maininstruction = u"To the view:\n'%s'\nhave been added filters:" %name else: maincontent = 'Filters were not added!\nFilters are not selected or are already added to the view!' maininstruction = u"To the view '%s':" %name header = 'Add multiple filters to current view' dialog = TaskDialog(header) dialog.TitleAutoPrefix = False dialog.AllowCancellation = True dialog.CommonButtons = TaskDialogCommonButtons.Ok; dialog.MainIcon = TaskDialogIcon.TaskDialogIconNone dialog.MainInstruction = maininstruction dialog.MainContent = maincontent if len(name) != 0 and IN[1][2] == 'Ok': dialog.Show()
Download script for Dynamo - Link
Comments
Post a Comment